JavaScript - JavaScript Debugging- WDD330📱
Debugging
This week we learned about testing and debugging in Javascript. There are different types of errors that we can detect, here are the types of errors you can encounter:
- Syntax Errors - errors that are detected by the compiler, a synstax error can be fix follow the correct systaxis and use tools for deterctions and debugging.
- Runtime Errors - errors that cause a program to terminate abnormally
- Logic Errors - occer when a program does not perform correctly and sometime this type of bugs are more difficult to resolve.
- The good think about JavaScript is that the community is big and you can resolve the bugs asking in places such StackOverFlow, GitHub, and see medium discussion to find the bug more efficient.
It's important to be able to recognize the errors during development process and minimize bugs during production, the error are a great way to distinguish and increase your performance as developer.
concole.log()
One debugging method is console.log()
. Using this allows us to display a value in
the console the possible error and what value is being broken.
a = 5;
b = 6;
x = a + b;
console.log(x);
you can see the value of "x" in the console accessing the developer tools inspect.
Breakpoints
We can set breakpoints and when our code runs into one, to evaluate the breakpoint that it will stop executing and we can see the current values. We can then resume the program.
Debugger Keyword
The debugger keyword is similar to settting a breakpoint. It stops the execution of the program where it is placed before continuing.
var x = 5 * 5;
debugger;
document.getElementById("demo").innerHTML = x;
Team Assignment
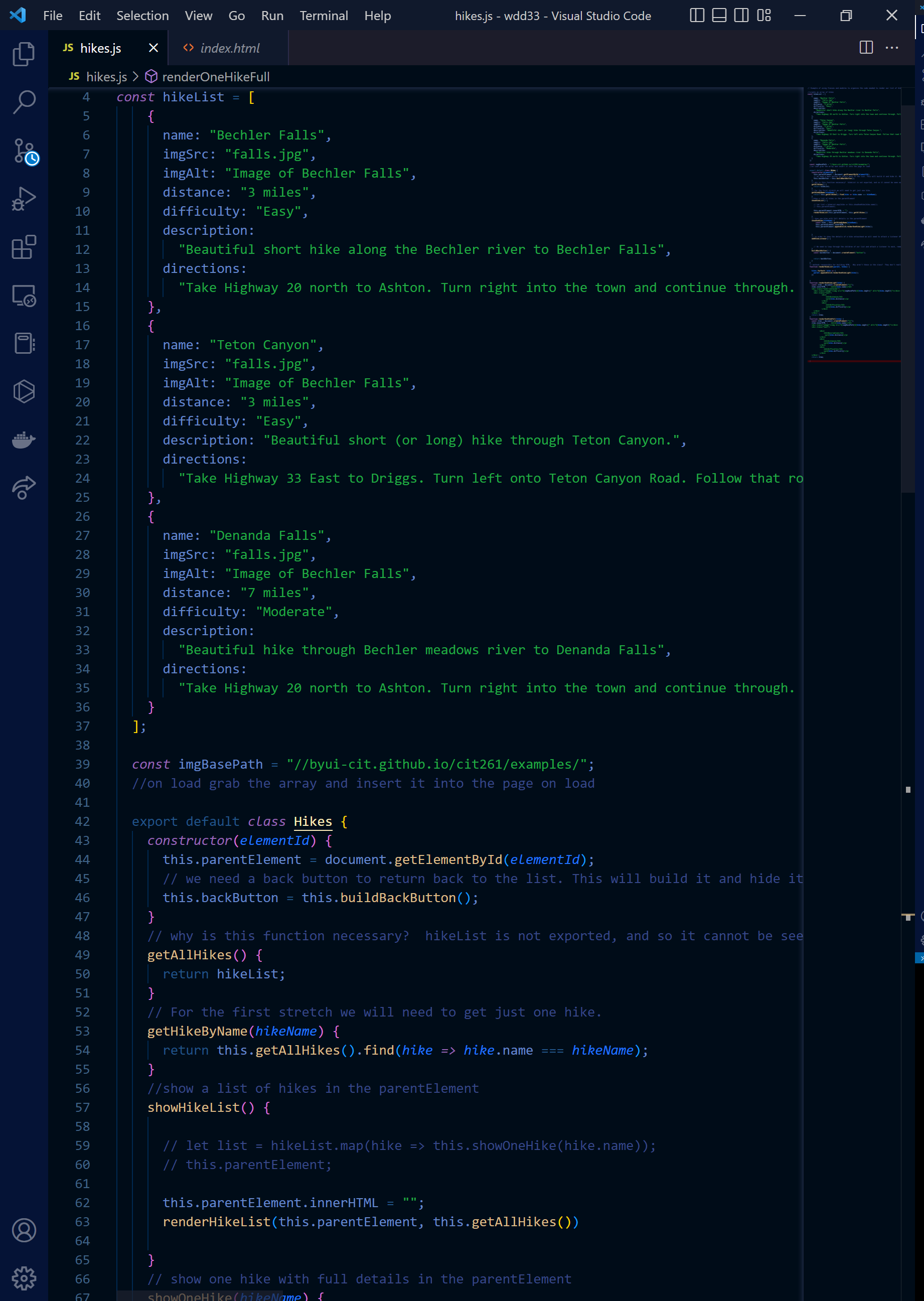
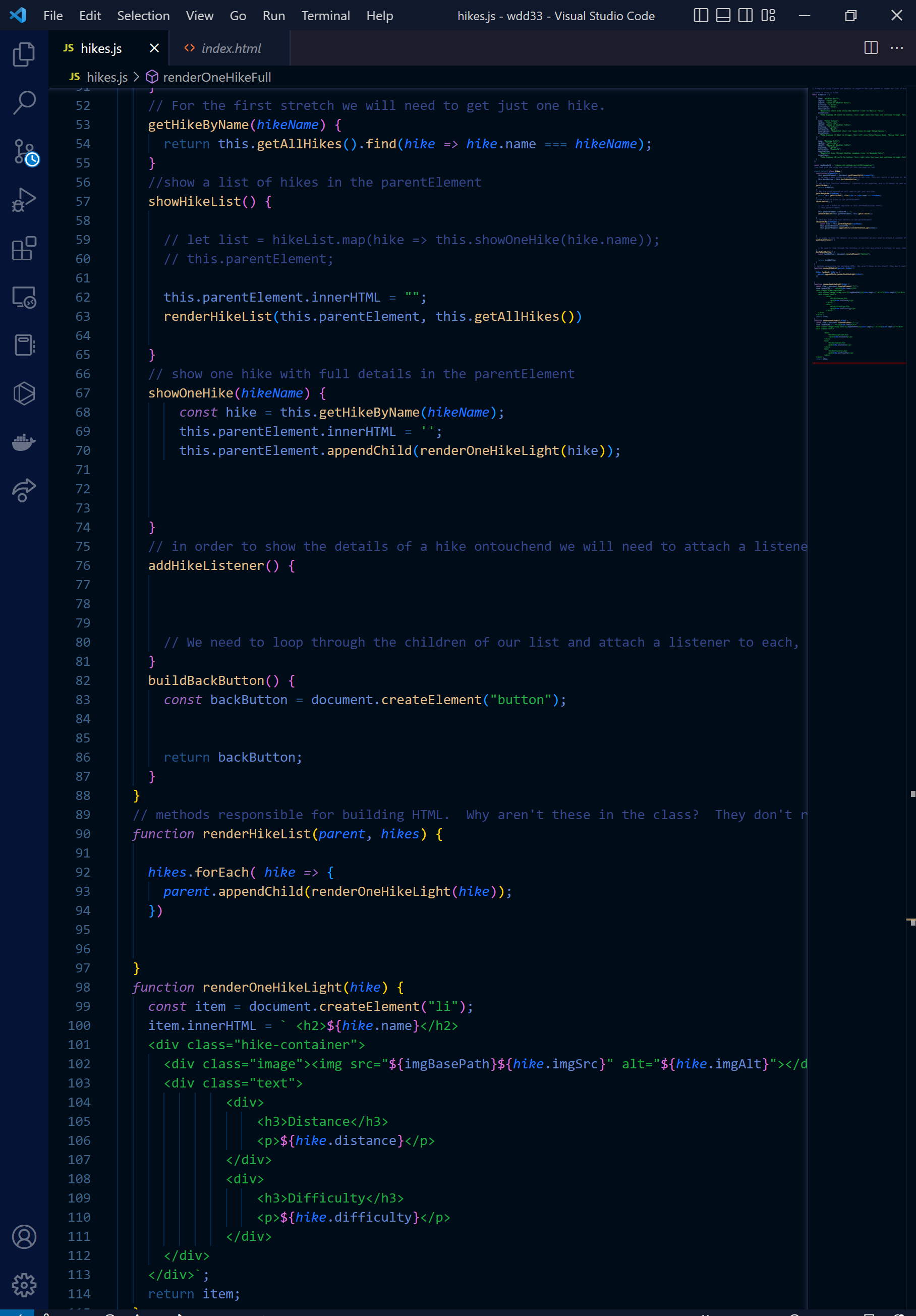
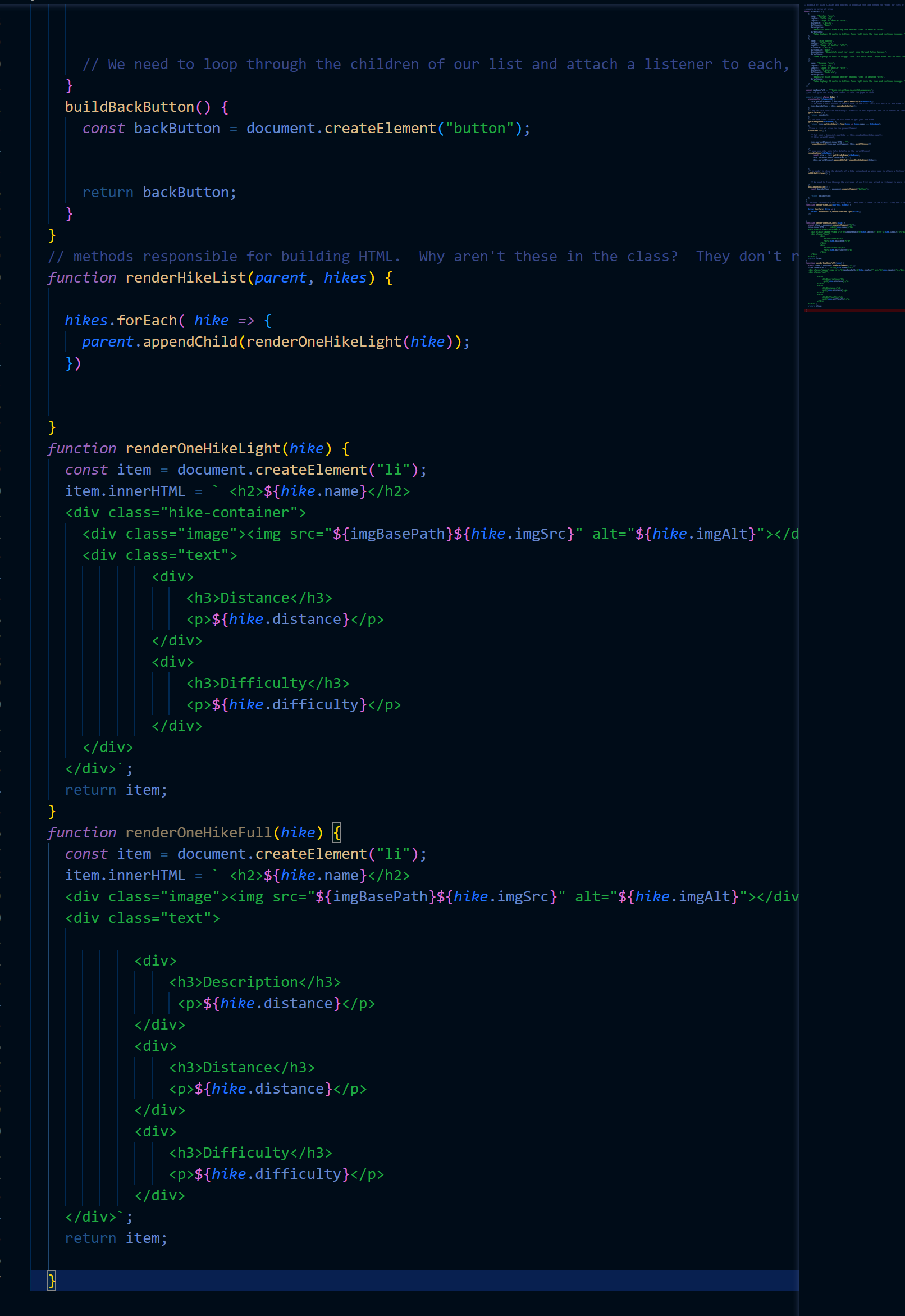
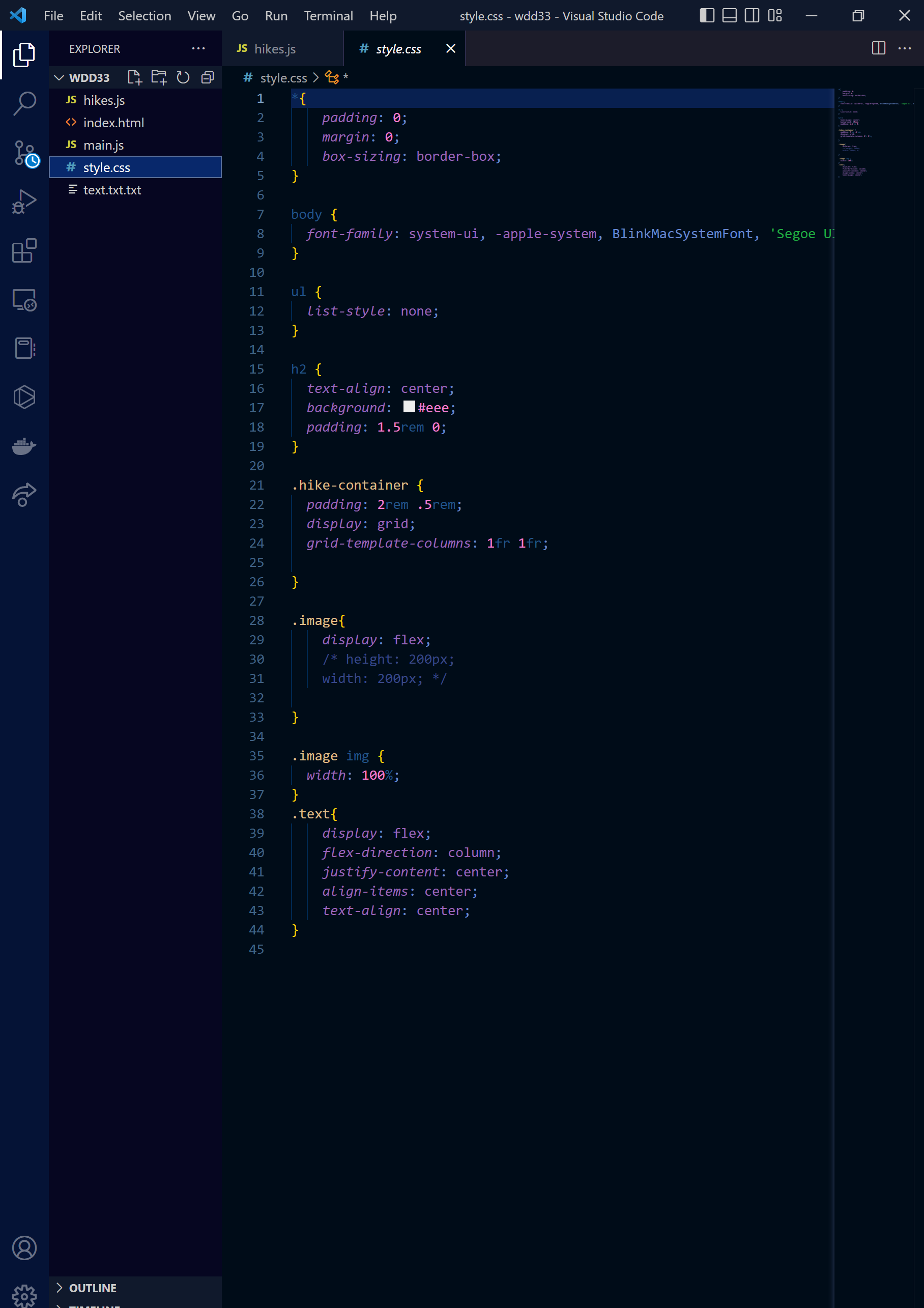
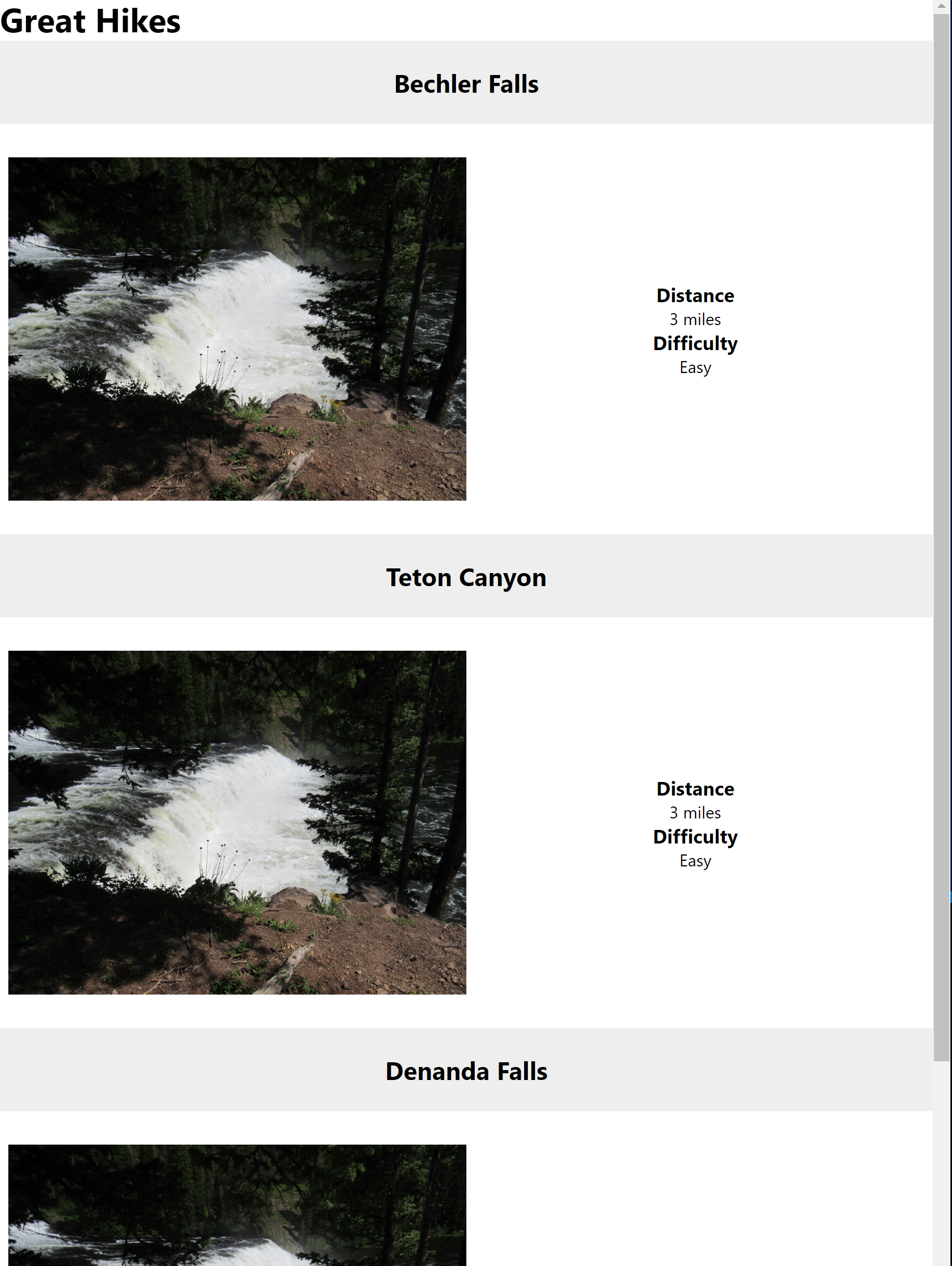
Reference
Forms: https://www.sitepoint.com/premium/books/javascript-novice-to-ninja-2nd-edition/read/8/ Object-Oriented Programming: https://www.sitepoint.com/premium/books/javascript-novice-to-ninja-2nd-edition/read/12/k01nwva7/Contact me
📧Email me at iodaniel@byui.edu
- Lesson 01: Notes
- Lesson 02:
- Lesson 03:
- Lesson 04:
- Lesson 05:
- Lesson 06:
- Lesson 07:
- Lesson 08:
- Lesson 09:
- Lesson 10:
- Lesson 11: